Overview
Prodly provides the Apex Class “DeploymentSchedulable” that you can call to schedule your deployments. Pass in the data set ID, and source and destination org IDs to schedule automatic deployments.
These steps provide one way to implement deployment scheduling:
- Open the Developer Console.
- Navigate to Debug > Open Execute Anonymous Window.
- Copy and paste this code.
Schedulable Service Methods
Provides wrappers to schedule deployments.
global with sharing class AppOpsDeploymentSchedulableService implements Schedulable {
global AppOpsDeploymentSchedulableService(PDRI.AppOpsWebServices.DeploymentServiceRequestV1 deploymentServiceRequest,
String destinationManagedInstanceId);
global AppOpsDeploymentSchedulableService(PDRI.AppOpsWebServices.DeploymentServiceRequestV1 deploymentServiceRequest,
List<String> destinationManagedInstanceIds);
global AppOpsDeploymentSchedulableService(String deploymentName,
String deploymentNotes,
String sourceOrgId,
String destinationOrgId,
String dataSetId,
String deploymentPlanId);
global AppOpsDeploymentSchedulableService(String deploymentName,
String deploymentNotes,
String sourceOrgId,
List<String> destinationOrgIds,
String dataSetId,
String deploymentPlanId);
}
Please, keep in mind that IDs should be specified in the 18-characters format. Otherwise, the scheduled job will fail.
Sample Code
Schedules a deployment.
//Data Set deployment to one destination every day at 8PM
PDRI.AppOpsDeploymentSchedulableService sch =
new PDRI.AppOpsDeploymentSchedulableService(
'Data Set Deployment',
'notes here',
'00DHn000002UdMdMAK',
'00DD7000000o50rMAA',
'a5jHn0000016GfjIAE',
null);
String cron = '0 0 20 * * ?';
System.schedule('One destination', cron, sch);
//Deployment plan deployment to 3 destinations every day at 8PM
PDRI.AppOpsDeploymentSchedulableService sch =
new PDRI.AppOpsDeploymentSchedulableService(
'Deployment Plan Deployment',
'notes here',
'00DHn000002UdMdMAK',
new List<String> {'00DD7000000o50rMAA', '00DD7000000o513MAA', '00DD7000000o4QiMAI'},
null,
'a5jHn0000016GfxOOO');
String cron = '0 0 20 * * ?';
System.schedule('Several destinations', cron, sch);
Check out more information on cron expressions in this SF article.
- Modify the code to use the appropriate constructor (refer to the screenshot below for choices) and schedule string.
- Execute the code.
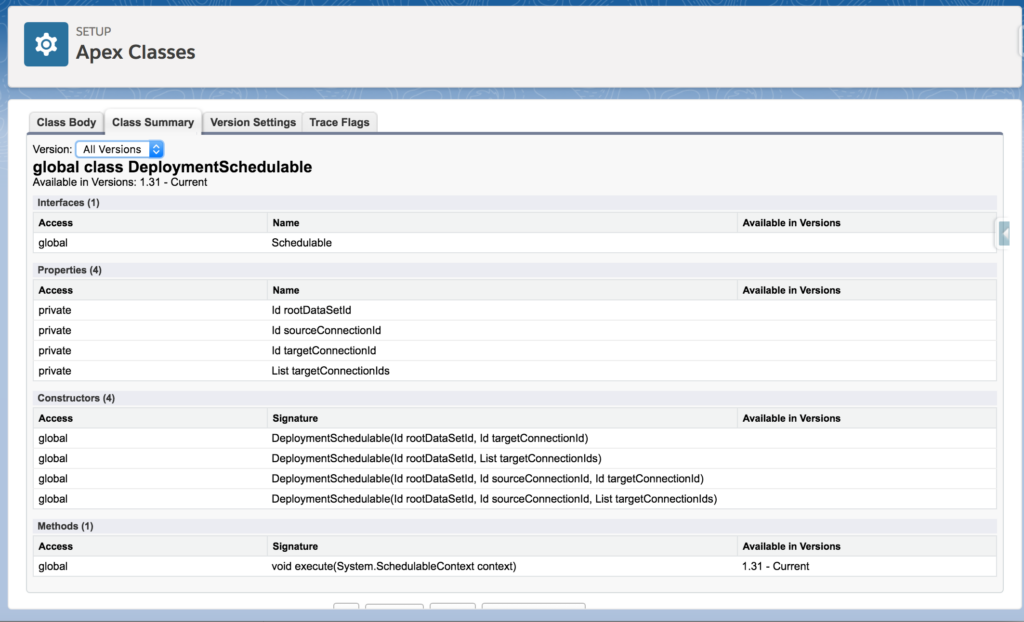